Framework
Dựa trên nhu cầu thực tế, chúng tôi cung cấp mẫu ví dụ giúp bạn tích hợp sản phẩm của chúng tôi vào dự án của bạn dễ dàng hơn.
React
React
The library for web and native user interfaces
Các bước thiết lập chung
- Tải source code
- Yêu cầu phiên bản Node.js >=15.0.0
- Cài đặt package theo câu lệnh dưới đây
npm install --save @goongmaps/goong-js
- Nhúng
link
vàscript
dưới đây vào thẻ<head>
của tệp HTML<script src='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.js'></script>
<link href='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.css' rel='stylesheet' />
Bản đồ
- Khởi tạo bản đồ
goongjs.accessToken = { YOUR_API_KEY };
var map = new goongjs.Map({
container: 'map',
style: 'https://tiles.goong.io/assets/goong_map_web.json',
center: [105.83991, 21.02800],
zoom: 9
});
goongjs.accessToken = { YOUR_API_KEY }: Nhập key mà bạn đã đăng ký vào đây
new goongjs.Map(): Khởi tạo bản đồ
center: Vị trí ban đầu khi bản đồ được tải lên
zoom: Độ phóng to của bản đồ
Autocomplete
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm add @goongmaps/goong-geocoder
- Nhúng
link
vàscript
dưới đây vào thẻ<body>
của tệp HTML<script src="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.css" rel="stylesheet" type="text/css" />
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.min.js"></script>
- Khởi tạo search box
map.addControl(
new GoongGeocoder({
accessToken: { YOUR_MAPTILES_KEY },
goongjs: goongjs
})
);
accessToken: { YOUR_MAPTILES_KEY }: Nhập key mà bạn đã đăng ký vào đây
new GoongGeocoder() : Khởi tạo search box
Đánh dấu
- Khởi tạo bản đồ
- Khởi tạo Marker trên bản đồ
- Khai báo locations
const [locations, setLocations] = useState([
{
key: '1',
coord: [107.58472, 16.46278],
},
{
key: '2',
coord: [108.03889, 12.66667],
},
...
])
locations: Một mảng bao gồm các đối tượng mà trong các đối tượng đó có chứa kinh độ và vĩ độ được dùng để đặt Marker trên bản đồ.
- Khởi tạo Marker
locations.map((location) => {
return new goongjs.Marker()
.setLngLat(location.coord)
.addTo(mapRef.current);
});
new goongjs.Marker(): Khởi tạo Marker.
setLngLat(): Truyền kinh độ vĩ độ cho Marker.
addTo(): Gán Marker lên bản đồ.
- Thêm Marker
const handleAddMarker = () => {
if (!lat || !lng) {
alert("Please enter locations")
} else if (selectLocation === null) {
setLng("")
setLat("")
setLocations(a => [...a, { key: (locations.length + 1).toString(), coord: [parseFloat(lng), parseFloat(lat)] }]);
} else {
setLng("")
setLat("")
setLocations((e) => {
const newLocations = [...e];
newLocations.splice(selectLocation, 1, { key: (selectLocation + 1).toString(), coord: [parseFloat(lng), parseFloat(lat)] });
return newLocations;
});
}
setSelectLocation(null)
};
Nếu người dùng không nhập kinh độ và vĩ độ thì sẽ có thông báo "Please enter locations", khi người dùng nhập kinh độ, vĩ độ vào ô Input, sẽ có hai trường hợp xảy ra:
Trường hợp 1: Người dùng thêm mới (selectLocation === null)
Trường hợp 2: Người dùng sửa (selectLocation !== null)
- Sửa Marker
const handleEdit = (key) => {
let index = locations.indexOf(key)
setLng(key.coord[0])
setLat(key.coord[1])
setSelectLocation(index)
}
Tham số truyền vào là key giúp cho người dùng lấy được vị trí của Marker cần sửa, sau đó dùng phương thức splice() để sửa (Trường hợp 2 đã nêu bên trên)
- Xoá Marker
const handleDelete = (index) => {
setLocations((e) => {
const newLocations = [...e];
newLocations.splice(index, 1);
return newLocations;
});
}
Tham số truyền vào là index giúp cho người dùng lấy được vị trí của Marker cần xoá, sau đó dùng phương thức splice() để xoá.
Dẫn đường
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm install @mapbox/polyline
npm install @goongmap/goong-sdk
npm install axios
- Các tham số cần thiết
- Khai báo biến
const [origin, setOrigin] = useState({});
const [destination, setDestination] = useState({});
const [startPoint, setStartPoint] = useState("")
const [endPoint, setEndPoint] = useState("")
"origin" và "destination" là Object chứa toạ độ của điểm đầu và điểm cuối
"startPoint" và "endPoint" là địa điểm mà người dùng nhập vào
- Kinh độ, vĩ độ của điểm bắt đầu
await axios.get(`${url}=${startPoint}&api_key=${process.env.REACT_APP_GOONG_MAP_KEY}`)
.then(res => {
const points = res.data;
setOrigin(points.results[0].geometry.location);
})
.catch(error => console.log(error));
- Kinh độ, vĩ độ của điểm kết thúc
await axios.get(`${url}=${endPoint}&api_key=${process.env.REACT_APP_GOONG_MAP_KEY}`)
.then(res => {
const points = res.data;
setDestination(points.results[0].geometry.location);
})
.catch(error => console.log(error));
Sử dụng Method: GET và URL: "https://rsapi.goong.io/geocode?address={YOUR_LOCATION}&api_key={YOUR_API_KEY}" để lấy toạ độ của điểm đầu và điểm kết thúc.
"YOUR_LOCATION" chính là "startPoint" và "endPoint" được khai báo ban đầu, sau khi người dùng nhập địa điểm cần tìm server sẽ trả về toạ độ, sau đó sẽ được gán vào hai object là "origin" và "destination"
- Truyền toạ độ điểm đầu và điểm cuối để vẽ tuyến đường qua 2 điểm
directionService
.getDirections({
// Truyền tham số vào đây
origin: `${origin.lat},${origin.lng}`,
destination: `${destination.lat},${destination.lng}`,
vehicle: 'car'
})
.send()
.then(function (response) {
// Dữ liệu của tuyến đường được trả về
var directions = response.body;
var route = directions.routes[0];
var geometry_string = route.overview_polyline.points;
var geoJSON = polyline.toGeoJSON(geometry_string);
// Bản đồ sẽ tiếp nhận dữ liệu
mapRef.current.addSource('route', {
'type': 'geojson',
'data': geoJSON
});
// Tuyến đường sẽ được vẽ trên bản đồ với các thông số dưới đây
mapRef.current.addLayer(
{
'id': 'route',
'type': 'line',
'source': 'route',
'layout': {
'line-join': 'round',
'line-cap': 'round'
},
'paint': {
'line-color': 'rgb(0, 153, 255)',
'line-width': 8
}
},
firstSymbolId
);
})
Tham khảo
Hiển thị bản đồ
Add a marker
Autocomplete
Dẫn đường
Vue2
Vue
An approachable, performant and versatile framework for building web user interfaces.
Các bước thiết lập chung
- Tải source code
- Yêu cầu phiên bản Node.js >=15.0.0
- Cài đặt package theo câu lệnh dưới đây
npm install --save @goongmaps/goong-js
- Nhúng
link
vàscript
dưới đây vào thẻ<head>
của tệpindex.html
<script src='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.js'></script>
<link href='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.css' rel='stylesheet' />
Bản đồ
- Khởi tạo bản đồ
goongjs.accessToken = { YOUR_API_KEY };
var map = new goongjs.Map({
container: 'map',
style: 'https://tiles.goong.io/assets/goong_map_web.json',
center: [105.83991, 21.02800],
zoom: 9
});
goongjs.accessToken = { YOUR_API_KEY }: Nhập key mà bạn đã đăng ký vào đây
new goongjs.Map(): Khởi tạo bản đồ
center: Vị trí ban đầu khi bản đồ được tải lên
zoom: Độ phóng to của bản đồ
Autocomplete
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm add @goongmaps/goong-geocoder
- Nhúng
link
vàscript
dưới đây vào thẻ<body>
của tệpindex.html
<script src="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.css" rel="stylesheet" type="text/css" />
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.min.js"></script>
- Khởi tạo search box
map.addControl(
new GoongGeocoder({
accessToken: { YOUR_MAPTILES_KEY },
goongjs: goongjs
})
);
accessToken: { YOUR_MAPTILES_KEY }: Nhập key mà bạn đã đăng ký vào đây
new GoongGeocoder() : Khởi tạo search box
Đánh dấu
- Khởi tạo bản đồ
- Khởi tạo Marker trên bản đồ
- Khai báo locations
locations = [
{
key: '1',
coord: [107.58472, 16.46278]
},
{
key: '2',
coord: [105.82556, 21.56750]
},
...
];
locations: Một mảng bao gồm các đối tượng mà trong các đối tượng đó có chứa kinh độ và vĩ độ được dùng để đặt Marker trên bản đồ.
- Khởi tạo Marker
initMarker(location) {
location.forEach(e => {
const marker = new goongjs.Marker()
.setLngLat(e.coord)
.addTo(this.map);
this.markers.push(marker)
})
},
new goongjs.Marker(): Khởi tạo Marker.
setLngLat(): Truyền kinh độ vĩ độ cho Marker.
addTo(): Gán Marker lên bản đồ.
- Thêm Marker
addMarker() {
if (!this.lng || !this.lat) {
alert('Please enter locations')
} else if (this.i === null) {
this.markers.forEach(marker => {
marker.remove();
});
console.log(`add`, this.i)
this.locations.push({ key: (this.locations.length + 1).toString(), coord: [parseFloat(this.lng), parseFloat(this.lat)] });
this.lat = null;
this.lng = null;
this.initMarker(this.locations);
} else {
this.markers.forEach(marker => {
marker.remove();
});
this.locations.splice(this.i, 1, { key: (this.i + 1).toString(), coord: [parseFloat(this.lng), parseFloat(this.lat)] });
this.initMarker(this.locations);
this.lat = null;
this.lng = null;
}
return this.i = null
},
Nếu người dùng không nhập kinh độ và vĩ độ thì sẽ có thông báo "Please enter locations", khi người dùng nhập kinh độ, vĩ độ vào ô Input, sẽ có hai trường hợp xảy ra:
Trường hợp 1: Người dùng thêm mới (tthis.i === null)
Trường hợp 2: Người dùng sửa (this.i !== null)
- Sửa Marker
handleEdit(item, index) {
this.lng = item.coord[0]
this.lat = item.coord[1];
this.i = index
},
Tham số truyền vào là index giúp cho người dùng lấy được vị trí của Marker cần sửa, sau đó dùng phương thức splice() để sửa (Trường hợp 2 đã nêu bên trên)
- Xoá Marker
handleDelete(item) {
let index = this.locations.indexOf(item)
this.i = index
if (this.markers) {
this.markers.forEach(marker => {
marker.remove();
});
}
this.locations.splice(this.i, 1);
this.initMarker(this.locations);
},
Tham số truyền vào là index giúp cho người dùng lấy được vị trí của Marker cần xoá, sau đó dùng phương thức splice() để xoá.
Dẫn đường
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm install @mapbox/polyline
npm install @goongmap/goong-sdk
npm install axios
- Các tham số cần thiết
- Khai báo biến
data(){
return {
startPoint: "",
endPoint: "",
}
}
"origin" và "destination" là Object chứa toạ độ của điểm đầu và điểm cuối
"startPoint" và "endPoint" là địa điểm mà người dùng nhập vào
- Kinh độ, vĩ độ của điểm bắt đầu và điểm kết thúc
const [originRes, destRes] = await Promise.all([
this.$myHttp.get(`${this.url}=${this.startPoint}&api_key=${this.GOONG_MAP_KEY}`),
this.$myHttp.get(`${this.url}=${this.endPoint}&api_key=${this.GOONG_MAP_KEY}`)
]);
const origin = originRes.data.results[0].geometry.location;
const destination = destRes.data.results[0].geometry.location;
this.direction({ origin, destination });
Sử dụng Method: GET và URL: "https://rsapi.goong.io/geocode?address={YOUR_LOCATION}&api_key={YOUR_API_KEY}" để lấy toạ độ của điểm đầu và điểm kết thúc.
"YOUR_LOCATION" chính là "startPoint" và "endPoint" được khai báo ban đầu, sau khi người dùng nhập địa điểm cần tìm server sẽ trả về toạ độ, sau đó sẽ được gán vào hai biến là "origin" và "destination"
- Truyền toạ độ điểm đầu và điểm cuối để vẽ tuyến đường qua 2 điểm
const originPlace = point.origin
const destinationPlace = point.destination
...
directionService
.getDirections({
// Truyền tham số vào đây
origin: `${originPlace.lat},${originPlace.lng}`,
destination: `${destinationPlace.lat},${destinationPlace.lng}`,
vehicle: 'car'
})
.send()
.then(function (response) {
// Dữ liệu của tuyến đường được trả về
var directions = response.body;
var route = directions.routes[0];
var geometry_string = route.overview_polyline.points;
var geoJSON = polyline.toGeoJSON(geometry_string);
// Bản đồ sẽ tiếp nhận dữ liệu
mapRef.current.addSource('route', {
'type': 'geojson',
'data': geoJSON
});
// Tuyến đường sẽ được vẽ trên bản đồ với các thông số dưới đây
mapRef.current.addLayer(
{
'id': 'route',
'type': 'line',
'source': 'route',
'layout': {
'line-join': 'round',
'line-cap': 'round'
},
'paint': {
'line-color': 'rgb(0, 153, 255)',
'line-width': 8
}
},
firstSymbolId
);
})
Tham khảo
Hiển thị bản đồ
Add a marker
Autocomplete
Dẫn đường
Vue3
Vue
An approachable, performant and versatile framework for building web user interfaces.
Các bước thiết lập chung
- Tải source code
- Yêu cầu phiên bản Node.js >=15.0.0
- Cài đặt package theo câu lệnh dưới đây
npm install --save @goongmaps/goong-js
- Nhúng
link
vàscript
dưới đây vào thẻ<head>
của tệp HTML<script src='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.js'></script>
<link href='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.css' rel='stylesheet' />
Bản đồ
- Khởi tạo bản đồ
goongjs.accessToken = { YOUR_API_KEY };
var map = new goongjs.Map({
container: 'map',
style: 'https://tiles.goong.io/assets/goong_map_web.json',
center: [105.83991, 21.02800],
zoom: 9
});
goongjs.accessToken = { YOUR_API_KEY }: Nhập key mà bạn đã đăng ký vào đây
new goongjs.Map(): Khởi tạo bản đồ
center: Vị trí ban đầu khi bản đồ được tải lên
zoom: Độ phóng to của bản đồ
Autocomplete
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm add @goongmaps/goong-geocoder
- Nhúng
link
vàscript
dưới đây vào thẻ<body>
của tệp HTML<script src="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.css" rel="stylesheet" type="text/css" />
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.min.js"></script>
- Khởi tạo search box
map.addControl(
new GoongGeocoder({
accessToken: { YOUR_MAPTILES_KEY },
goongjs: goongjs
})
);
accessToken: { YOUR_MAPTILES_KEY }: Nhập key mà bạn đã đăng ký vào đây
new GoongGeocoder() : Khởi tạo search box
Đánh dấu
- Khởi tạo bản đồ
- Khởi tạo Marker trên bản đồ
- Khai báo locations
const locations = ref([
{
key: '1',
coord: [107.58472, 16.46278]
},
{
key: '2',
coord: [105.82556, 21.56750]
},
...
])
locations: Một mảng bao gồm các đối tượng mà trong các đối tượng đó có chứa kinh độ và vĩ độ được dùng để đặt Marker trên bản đồ.
- Khởi tạo Marker
function initMarker(locations) {
locations.forEach(e => {
var popup = new goongjs.Popup({ offset: 0 }).setText(
'Goong Map'
);
// Tuỳ chỉnh hình dạng của Marker
var el = document.createElement('div');
el.style.backgroundColor = "red"
el.style.width = '25px';
el.style.height = '25px';
el.style.borderRadius = "50%"
new goongjs.Marker(el).setLngLat(e.coord).setPopup(popup).addTo(map.value);
const marker = new goongjs.Marker(el)
.setLngLat(e.coord)
.addTo(map.value);
markers.push(marker)
})
}
new goongjs.Marker(): Khởi tạo Marker.
setLngLat(): Truyền kinh độ vĩ độ cho Marker.
addTo(): Gán Marker lên bản đồ.
- Thêm Marker
function addMarker() {
if (!lng.value || !lat.value) {
alert('Please enter locations')
} else if (i.value === null) {
locations.value.push({ key: (locations.value.length + 1).toString(), coord: [lng.value, lat.value] });
initMarker(locations.value)
lat.value = null;
lng.value = null
} else {
markers.forEach(marker => {
marker.remove();
});
locations.value.splice(i.value, 1, { key: (i.value + 1).toString(), coord: [lng.value, lat.value] });
initMarker(locations.value)
lat.value = null;
lng.value = null;
}
return i.value = null
}
Nếu người dùng không nhập kinh độ và vĩ độ thì sẽ có thông báo "Please enter locations", khi người dùng nhập kinh độ, vĩ độ vào ô Input, sẽ có hai trường hợp xảy ra:
Trường hợp 1: Người dùng thêm mới (i.value === null)
Trường hợp 2: Người dùng sửa (i.value !== null)
- Sửa Marker
function handleEdit(item, index) {
lng.value = item.coord[0]
lat.value = item.coord[1];
i.value = index
}
Tham số truyền vào là key giúp cho người dùng lấy được vị trí của Marker cần sửa, sau đó dùng phương thức splice() để sửa (Trường hợp 2 đã nêu bên trên)
- Xoá Marker
function handleDelete(index) {
if (markers) {
markers.forEach(marker => {
marker.remove();
});
}
locations.value.splice(index, 1);
initMarker(locations.value)
}
Tham số truyền vào là index giúp cho người dùng lấy được vị trí của Marker cần xoá, sau đó dùng phương thức splice() để xoá.
Dẫn đường
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm install @mapbox/polyline
npm install @goongmap/goong-sdk
npm install axios
- Các tham số cần thiết
- Khai báo biến
const startPoint = ref("")
const endPoint = ref("")
"origin" và "destination" là Object chứa toạ độ của điểm đầu và điểm cuối
"startPoint" và "endPoint" là địa điểm mà người dùng nhập vào
- Kinh độ, vĩ độ của điểm bắt đầu và điểm kết thúc
const [originRes, destRes] = await Promise.all([
axios.get(`${url}=${startPoint.value}&api_key=${GOONG_MAP_KEY}`),
axios.get(`${url}=${endPoint.value}&api_key=${GOONG_MAP_KEY}`)
]);
const origin = originRes.data.results[0].geometry.location;
const destination = destRes.data.results[0].geometry.location;
direction({ origin, destination });
Sử dụng Method: GET và URL: "https://rsapi.goong.io/geocode?address={YOUR_LOCATION}&api_key={YOUR_API_KEY}" để lấy toạ độ của điểm đầu và điểm kết thúc.
"YOUR_LOCATION" chính là "startPoint" và "endPoint" được khai báo ban đầu, sau khi người dùng nhập địa điểm cần tìm server sẽ trả về toạ độ, sau đó sẽ được gán vào hai biến là "origin" và "destination"
- Truyền toạ độ điểm đầu và điểm cuối để vẽ tuyến đường qua 2 điểm
const originPlace = point.origin
const destinationPlace = point.destination
...
directionService
.getDirections({
// Truyền tham số vào đây
origin: `${originPlace.lat},${originPlace.lng}`,
destination: `${destinationPlace.lat},${destinationPlace.lng}`,
vehicle: 'car'
})
.send()
.then(function (response) {
// Dữ liệu của tuyến đường được trả về
var directions = response.body;
var route = directions.routes[0];
var geometry_string = route.overview_polyline.points;
var geoJSON = polyline.toGeoJSON(geometry_string);
// Bản đồ sẽ tiếp nhận dữ liệu
mapRef.current.addSource('route', {
'type': 'geojson',
'data': geoJSON
});
// Tuyến đường sẽ được vẽ trên bản đồ với các thông số dưới đây
mapRef.current.addLayer(
{
'id': 'route',
'type': 'line',
'source': 'route',
'layout': {
'line-join': 'round',
'line-cap': 'round'
},
'paint': {
'line-color': 'rgb(0, 153, 255)',
'line-width': 8
}
},
firstSymbolId
);
})
Tham khảo
Hiển thị bản đồ
Add a marker
Autocomplete
Dẫn đường
Angular
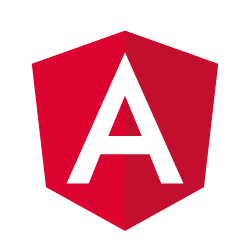
Angular
Deliver web apps with confidence
Các bước thiết lập chung
- Tải source code
- Yêu cầu phiên bản Node.js >=15.0.0
- Cài đặt package theo câu lệnh dưới đây
npm install --save @goongmaps/goong-js
- Nhúng
link
vàscript
dưới đây vào thẻ<head>
của tệpindex.html
<script src='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.js'></script>
<link href='https://cdn.jsdelivr.net/npm/@goongmaps/goong-js/dist/goong-js.css' rel='stylesheet' />
Bản đồ
- Khởi tạo bản đồ
goongjs.accessToken = { YOUR_API_KEY };
var map = new goongjs.Map({
container: 'map',
style: 'https://tiles.goong.io/assets/goong_map_web.json',
center: [105.83991, 21.02800],
zoom: 9
});
goongjs.accessToken = { YOUR_API_KEY }: Nhập key mà bạn đã đăng ký vào đây
new goongjs.Map(): Khởi tạo bản đồ
center: Vị trí ban đầu khi bản đồ được tải lên
zoom: Độ phóng to của bản đồ
Autocomplete
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm add @goongmaps/goong-geocoder
- Nhúng
link
vàscript
dưới đây vào thẻ<body>
của tệpindex.html
<script src="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/@goongmaps/goong-geocoder@1.1.1/dist/goong-geocoder.css" rel="stylesheet" type="text/css" />
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.min.js"></script>
- Khởi tạo search box
map.addControl(
new GoongGeocoder({
accessToken: { YOUR_MAPTILES_KEY },
goongjs: goongjs
})
);
accessToken: { YOUR_MAPTILES_KEY }: Nhập key mà bạn đã đăng ký vào đây
new GoongGeocoder() : Khởi tạo search box
Đánh dấu
- Khởi tạo bản đồ
- Khởi tạo Marker trên bản đồ
- Khai báo locations
locations = [
{
key: '1',
coord: [107.58472, 16.46278]
},
{
key: '2',
coord: [105.82556, 21.56750]
},
...
];
locations: Một mảng bao gồm các đối tượng mà trong các đối tượng đó có chứa kinh độ và vĩ độ được dùng để đặt Marker trên bản đồ.
- Khởi tạo Marker
initMarker(locations: any) {
locations.forEach((e: any) => {
const marker = new goongjs.Marker()
.setLngLat(e.coord)
.addTo(this.map);
this.markers.push(marker);
});
}
new goongjs.Marker(): Khởi tạo Marker.
setLngLat(): Truyền kinh độ vĩ độ cho Marker.
addTo(): Gán Marker lên bản đồ.
- Thêm Marker
addMarker() {
if (!this.lng || !this.lat) {
alert("Please enter locations")
} else if (this.index === null) {
this.markers.forEach(marker => {
marker.remove();
});
this.markers = [];
this.locations.push({ key: (this.locations.length + 1).toString(), coord: [parseInt(this.lng), parseInt(this.lat)] });
this.lat = null;
this.lng = null;
this.initMarker(this.locations);
} else {
this.markers.forEach(marker => {
marker.remove();
});
this.markers = [];
this.locations.splice(this.index, 1, { key: (this.index + 1).toString(), coord: [parseFloat(this.lng), parseFloat(this.lat)] });
this.lat = null;
this.lng = null;
this.initMarker(this.locations);
}
this.index = null
}
Nếu người dùng không nhập kinh độ và vĩ độ thì sẽ có thông báo "Please enter locations", khi người dùng nhập kinh độ, vĩ độ vào ô Input, sẽ có hai trường hợp xảy ra:
Trường hợp 1: Người dùng thêm mới (this.index === null)
Trường hợp 2: Người dùng sửa (this.index !== null)
- Sửa Marker
handleEdit(locations: any): void {
let i: number = this.locations.indexOf(locations)
this.index = i
this.lng = locations.coord[0]
this.lat = locations.coord[1]
console.log(this.index)
}
Tham số truyền vào là key giúp cho người dùng lấy được vị trí của Marker cần sửa, sau đó dùng phương thức splice() để sửa (Trường hợp 2 đã nêu bên trên)
- Xoá Marker
handleDelete(locations: any) {
let i: number = this.locations.indexOf(locations)
this.index = i
if (this.markers) {
this.markers.forEach(marker => {
marker.remove();
});
}
this.locations.splice(this.index, 1);
this.initMarker(this.locations);
}
Tham số truyền vào là index giúp cho người dùng lấy được vị trí của Marker cần xoá, sau đó dùng phương thức splice() để xoá.
Dẫn đường
- Khởi tạo bản đồ
- Cài đặt thêm package theo câu lệnh dưới đây
npm install @mapbox/polyline
npm install @goongmap/goong-sdk
npm install axios
- Các tham số cần thiết
- Khai báo biến
startPoint: string = "";
endPoint: string = "";
"origin" và "destination" là Object chứa toạ độ của điểm đầu và điểm cuối
"startPoint" và "endPoint" là địa điểm mà người dùng nhập vào
- Kinh độ, vĩ độ của điểm bắt đầu và điểm kết thúc
const [originRes, destRes] = await Promise.all([
axios.get(`${this.url}=${this.startPoint}&api_key=${environment.ANGULAR_APP_GOONG_MAP_KEY}`),
axios.get(`${this.url}=${this.endPoint}&api_key=${environment.ANGULAR_APP_GOONG_MAP_KEY}`)
]);
const origin = originRes.data.results[0].geometry.location;
const destination = destRes.data.results[0].geometry.location;
this.direction({ origin, destination });
Sử dụng Method: GET và URL: "https://rsapi.goong.io/geocode?address={YOUR_LOCATION}&api_key={YOUR_API_KEY}" để lấy toạ độ của điểm đầu và điểm kết thúc.
"YOUR_LOCATION" chính là "startPoint" và "endPoint" được khai báo ban đầu, sau khi người dùng nhập địa điểm cần tìm server sẽ trả về toạ độ, sau đó sẽ được gán vào hai biến là "origin" và "destination"
- Truyền toạ độ điểm đầu và điểm cuối để vẽ tuyến đường qua 2 điểm
const originPlace = point.origin
const destinationPlace = point.destination
...
directionService
.getDirections({
// Truyền tham số vào đây
origin: `${originPlace.lat},${originPlace.lng}`,
destination: `${destinationPlace.lat},${destinationPlace.lng}`,
vehicle: 'car'
})
.send()
.then(function (response:any):void {
// Dữ liệu của tuyến đường được trả về
var directions = response.body;
var route = directions.routes[0];
var geometry_string = route.overview_polyline.points;
var geoJSON = polyline.toGeoJSON(geometry_string);
// Bản đồ sẽ tiếp nhận dữ liệu
map.addSource('route', {
'type': 'geojson',
'data': geoJSON
});
// Tuyến đường sẽ được vẽ trên bản đồ với các thông số dưới đây
map.addLayer(
{
'id': 'route',
'type': 'line',
'source': 'route',
'layout': {
'line-join': 'round',
'line-cap': 'round'
},
'paint': {
'line-color': 'rgb(0, 153, 255)',
'line-width': 8
}
},
firstSymbolId
);
})
Tham khảo
Hiển thị bản đồ
Add a marker
Autocomplete
Dẫn đường